π $match
The $match operator is used in conjunction with the aggregation framework to filter documents in a collection. It takes a document as input and returns a new document containing only the documents that match the specified criteria. The syntax for the $match operator is as follows:
{ $match: { <expression>: <value> } }
Expressionsβ
The <expression>
portion of the $match operator can be any valid MongoDB expression. This includes :
- Comparison operators:
eq
,neq
,gte
,lte
,gt
,lt
,in
,nin
,exists
- Regular expressions: regex
- Logical operators: and, or, not
- Subdocuments and arrays:
{ field: <value> }, [ <item>, <item>, ... ]
Matching book documentsβ
- Atlas UI
- MongoDB Shell
First, make sure you select the books
collection in the Atlas UI.
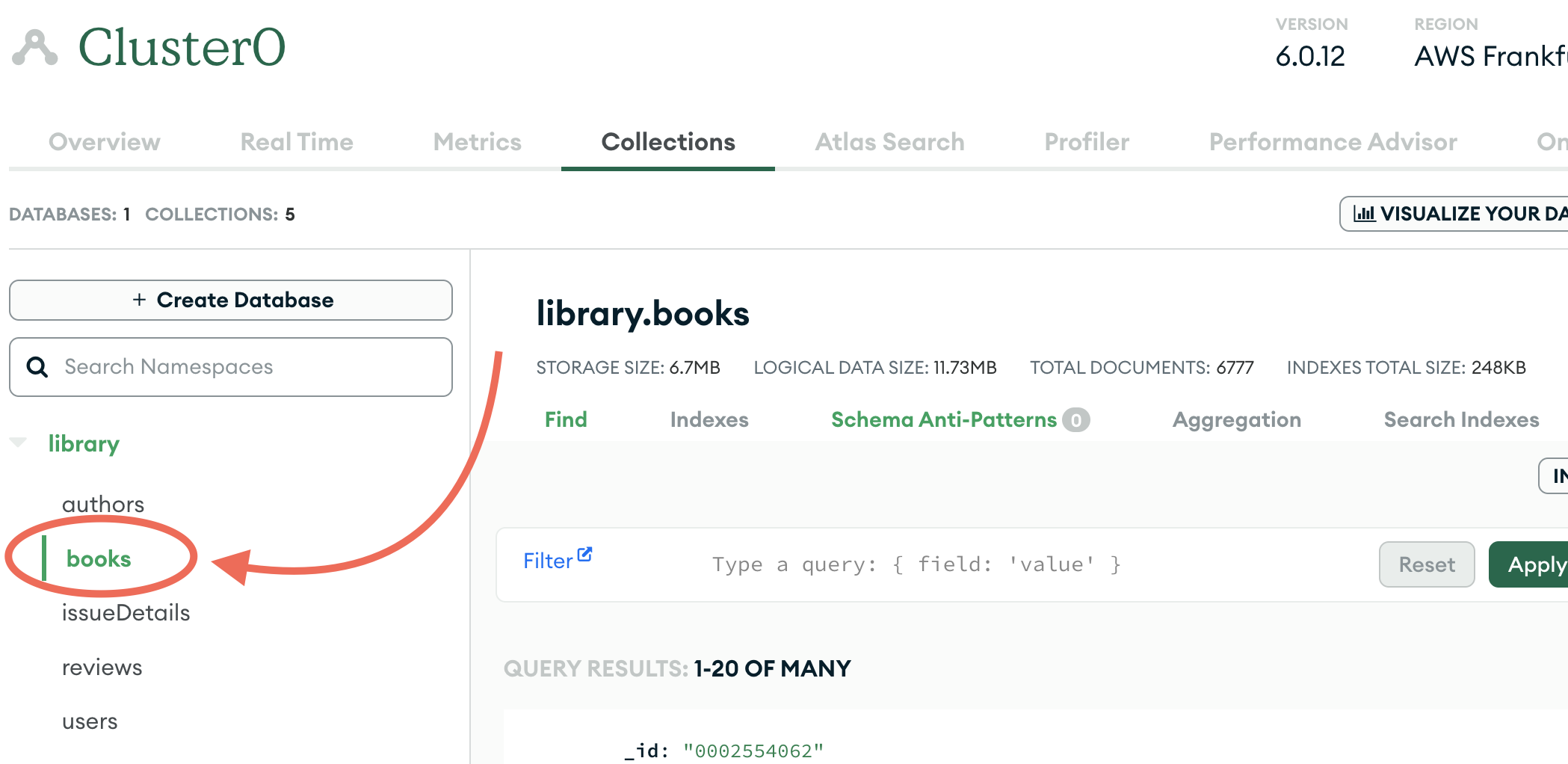
Then, navigate to the Aggregation
tab and click Add Stage
.
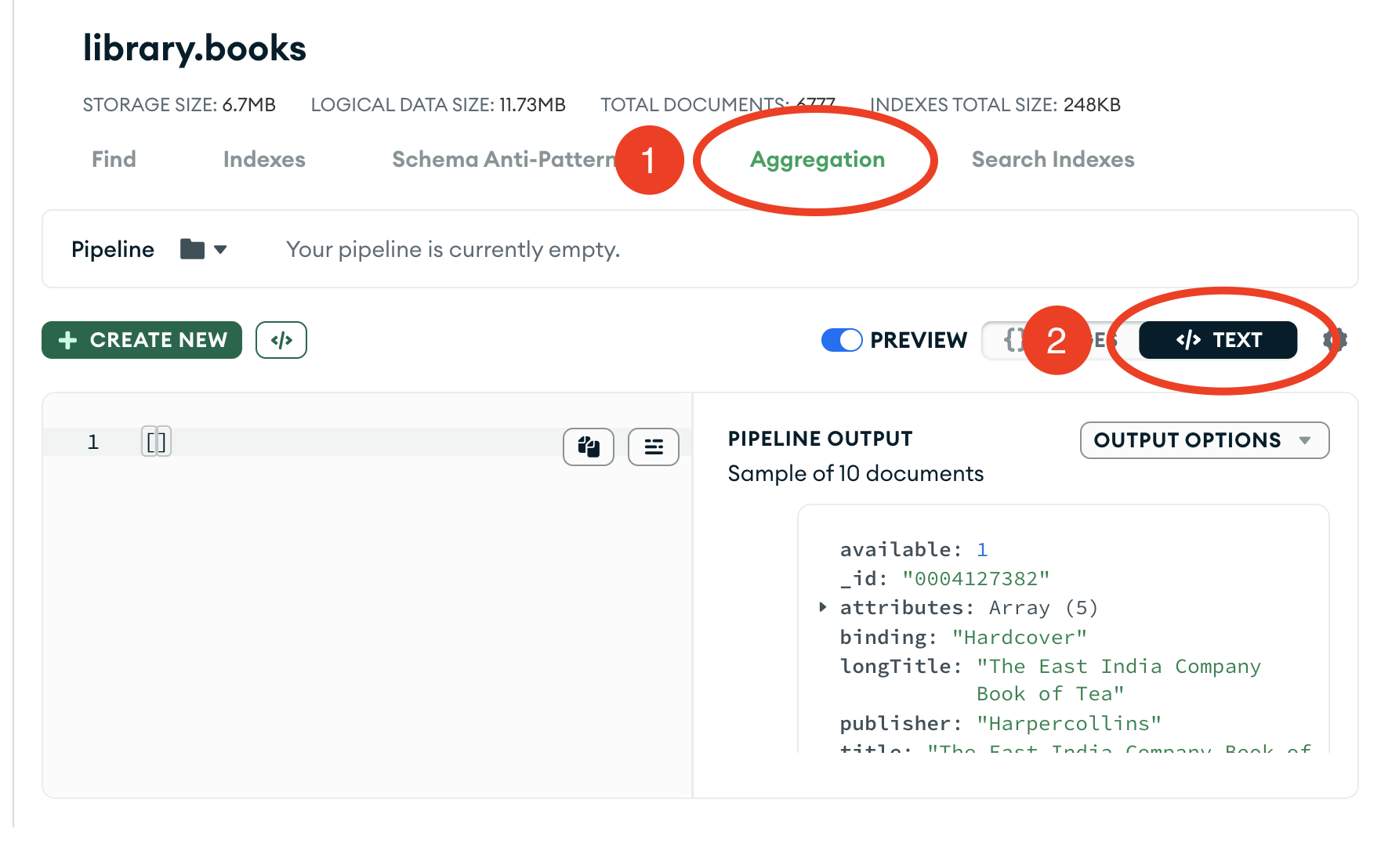
Say we want all the books from the year 2010. We can add a $match
stage to filter the documents from the books collection:
[
{
$match: { year: 2010 }
}
]
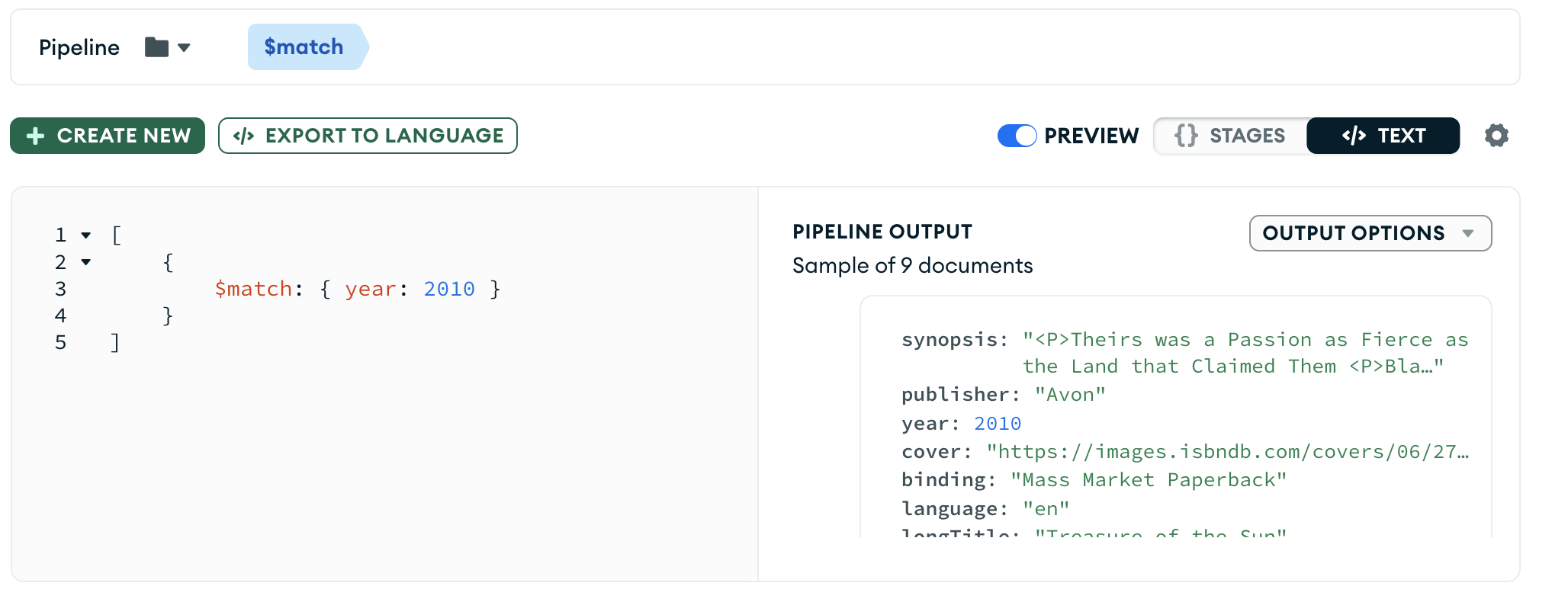
Say we want all the books from 2010. We'll write:
db.books.aggregate([{$match: {year: 2010}}])
π Return all the books
that have exactly 100 pages.
Answer
- Atlas UI
- MongoDB Shell
[
{
$match: { pages: 100 }
}
]
db.books.aggregate([{$match: {pages: 100}}])
ANDβ
If we need to add more conditions using AND, we can do it with the $and
operator.
If we want all the books with 100 pages with exactly totalInventory
2 we can use an $and
operator. This takes and array of documents with all the conditions that should be true for the AND to succeed:
- Atlas UI
- MongoDB Shell
[
{
$match: {
$and: [
{ pages: 100 },
{ totalInventory: 2 }
]
}
}
]
db.books.aggregate([{$match: {$and: [{pages: 100}, {totalInventory: 2}]}}])
The pseudo-code for this would be something like:
IF pages == 100 AND totalInventory == 2 {
return matching docs
}
π Return all the books
from 2015 that have exactly 100 pages.
Answer
- Atlas UI
- MongoDB Shell
[
{
$match: {
$and: [
{ pages: 100 },
{ year: 2015 }
]
}
}
]
db.books.aggregate([{$match: {$and: [{pages: 100}, {year: 2015}]}}])
π How many are they?
Answer
- Atlas UI
- MongoDB Shell
[
{
$match: {
$and: [
{ pages: 100 },
{ year: 2015 }
]
}
},
{
$count: "books_count"
}
]
db.books.aggregate([{$match: {$and: [{pages: 100}, {year: 2015}]}}]).itcount()
Shorthand ANDβ
We can do an implicit AND just passing a document with all the conditions (instead of an array of documents):
- Atlas UI
- MongoDB Shell
[
{
$match: {pages: 100, totalInventory: 2}
}
]
db.books.aggregate([{$match: {pages: 100, totalInventory: 2}}])
π Return all the books
from 2015 that have exactly 100 pages, using the simple $and notation
Answer
- Atlas UI
- MongoDB Shell
[
{
$match: {pages: 100, year: 2015}
}
]
db.books.aggregate([{$match: {pages: 100, year: 2015}}])